Artificial Intelligence
Optimizing LLM Deployment: vLLM PagedAttention and the Future of Efficient AI Serving
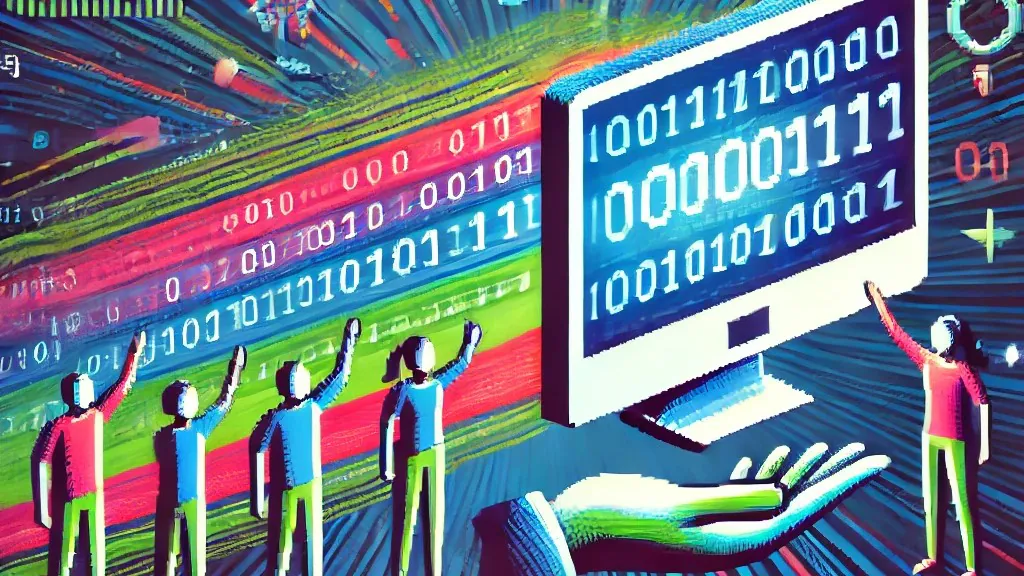
Large Language Models (LLMs) deploying on real-world applications presents unique challenges, particularly in terms of computational resources, latency, and cost-effectiveness. In this comprehensive guide, we'll explore the landscape of LLM serving, with a particular focus on vLLM (vector Language Model), a solution that's reshaping the way we deploy and interact with these powerful models.
The Challenges of Serving Large Language Models
Before diving into specific solutions, let's examine the key challenges that make LLM serving a complex task:
Computational Resources
LLMs are notorious for their enormous parameter counts, ranging from billions to hundreds of billions. For instance, GPT-3 boasts 175 billion parameters, while more recent models like GPT-4 are estimated to have even more. This sheer size translates to significant computational requirements for inference.
Example:
Consider a relatively modest LLM with 13 billion parameters, such as LLaMA-13B. Even this model requires:
– Approximately 26 GB of memory just to store the model parameters (assuming 16-bit precision)
– Additional memory for activations, attention mechanisms, and intermediate computations
– Substantial GPU compute power for real-time inference
Latency
In many applications, such as chatbots or real-time content generation, low latency is crucial for a good user experience. However, the complexity of LLMs can lead to significant processing times, especially for longer sequences.
Example:
Imagine a customer service chatbot powered by an LLM. If each response takes several seconds to generate, the conversation will feel unnatural and frustrating for users.
Cost
The hardware required to run LLMs at scale can be extremely expensive. High-end GPUs or TPUs are often necessary, and the energy consumption of these systems is substantial.
Example:
Running a cluster of NVIDIA A100 GPUs (often used for LLM inference) can cost thousands of dollars per day in cloud computing fees.
Traditional Approaches to LLM Serving
Before exploring more advanced solutions, let's briefly review some traditional approaches to serving LLMs:
Simple Deployment with Hugging Face Transformers
The Hugging Face Transformers library provides a straightforward way to deploy LLMs, but it's not optimized for high-throughput serving.
Example code:
from transformers import AutoModelForCausalLM, AutoTokenizer import torch model_name = "meta-llama/Llama-2-13b-hf" model = AutoModelForCausalLM.from_pretrained(model_name, device_map="auto") tokenizer = AutoTokenizer.from_pretrained(model_name) def generate_text(prompt, max_length=100): inputs = tokenizer(prompt, return_tensors="pt").to(model.device) outputs = model.generate(**inputs, max_length=max_length) return tokenizer.decode(outputs[0], skip_special_tokens=True) print(generate_text("The future of AI is"))
While this approach works, it's not suitable for high-traffic applications due to its inefficient use of resources and lack of optimizations for serving.
Using TorchServe or Similar Frameworks
Frameworks like TorchServe provide more robust serving capabilities, including load balancing and model versioning. However, they still don't address the specific challenges of LLM serving, such as efficient memory management for large models.
Understanding Memory Management in LLM Serving
Efficient memory management is critical for serving large language models (LLMs) due to the extensive computational resources required. The following images illustrate various aspects of memory management, which are integral to optimizing LLM performance.
Segmented vs. Paged Memory
These two diagrams compare segmented memory and paged memory management techniques, commonly used in operating systems (OS).
- Segmented Memory: This technique divides memory into different segments, each corresponding to a different program or process. For instance, in an LLM serving context, different segments might be allocated to various components of the model, such as tokenization, embedding, and attention mechanisms. Each segment can grow or shrink independently, providing flexibility but potentially leading to fragmentation if segments are not managed properly.
- Paged Memory: Here, memory is divided into fixed-size pages, which are mapped onto physical memory. Pages can be swapped in and out as needed, allowing for efficient use of memory resources. In LLM serving, this can be crucial for managing the large amounts of memory required for storing model weights and intermediate computations.
Memory Management in OS vs. vLLM
This image contrasts traditional OS memory management with the memory management approach used in vLLM.
- OS Memory Management: In traditional operating systems, processes (e.g., Process A and Process B) are allocated pages of memory (Page 0, Page 1, etc.) in physical memory. This allocation can lead to fragmentation over time as processes request and release memory.
- vLLM Memory Management: The vLLM framework uses a Key-Value (KV) cache to manage memory more efficiently. Requests (e.g., Request A and Request B) are allocated blocks of the KV cache (KV Block 0, KV Block 1, etc.). This approach helps minimize fragmentation and optimizes memory usage, allowing for faster and more efficient model serving.
Attention Mechanism in LLMs
The attention mechanism is a fundamental component of transformer models, which are commonly used for LLMs. This diagram illustrates the attention formula and its components:
- Query (Q): A new token in the decoder step or the last token that the model has seen.
- Key (K): Previous context that the model should attend to.
- Value (V): Weighted sum over the previous context.
The formula calculates the attention scores by taking the dot product of the query with the keys, scaling by the square root of the key dimension, applying a softmax function, and finally taking the dot product with the values. This process allows the model to focus on relevant parts of the input sequence when generating each token.
Serving Throughput Comparison
This image presents a comparison of serving throughput between different frameworks (HF, TGI, and vLLM) using LLaMA models on different hardware setups.
- LLaMA-13B, A100-40GB: vLLM achieves 14x – 24x higher throughput than HuggingFace Transformers (HF) and 2.2x – 2.5x higher throughput than HuggingFace Text Generation Inference (TGI).
- LLaMA-7B, A10G: Similar trends are observed, with vLLM significantly outperforming both HF and TGI.
vLLM: A New LLM Serving Architecture
vLLM, developed by researchers at UC Berkeley, represents a significant leap forward in LLM serving technology. Let's explore its key features and innovations:
PagedAttention
At the heart of vLLM lies PagedAttention, a novel attention algorithm inspired by virtual memory management in operating systems. Here's how it works:
– Key-Value (KV) Cache Partitioning: Instead of storing the entire KV cache contiguously in memory, PagedAttention divides it into fixed-size blocks.
– Non-Contiguous Storage: These blocks can be stored non-contiguously in memory, allowing for more flexible memory management.
– On-Demand Allocation: Blocks are allocated only when needed, reducing memory waste.
– Efficient Sharing: Multiple sequences can share blocks, enabling optimizations for techniques like parallel sampling and beam search.
Illustration:
“`
Traditional KV Cache:
[Token 1 KV][Token 2 KV][Token 3 KV]…[Token N KV]
(Contiguous memory allocation)
PagedAttention KV Cache:
[Block 1] -> Physical Address A
[Block 2] -> Physical Address C
[Block 3] -> Physical Address B
…
(Non-contiguous memory allocation)
“`
This approach significantly reduces memory fragmentation and allows for much more efficient use of GPU memory.
Continuous Batching
vLLM implements continuous batching, which dynamically processes requests as they arrive, rather than waiting to form fixed-size batches. This leads to lower latency and higher throughput.
Example:
Imagine a stream of incoming requests:
“`
Time 0ms: Request A arrives
Time 10ms: Start processing Request A
Time 15ms: Request B arrives
Time 20ms: Start processing Request B (in parallel with A)
Time 25ms: Request C arrives
…
“`
With continuous batching, vLLM can start processing each request immediately, rather than waiting to group them into predefined batches.
Efficient Parallel Sampling
For applications that require multiple output samples per prompt (e.g., creative writing assistants), vLLM's memory sharing capabilities shine. It can generate multiple outputs while reusing the KV cache for shared prefixes.
Example code using vLLM:
from vllm import LLM, SamplingParams llm = LLM(model="meta-llama/Llama-2-13b-hf") prompts = ["The future of AI is"] # Generate 3 samples per prompt sampling_params = SamplingParams(n=3, temperature=0.8, max_tokens=100) outputs = llm.generate(prompts, sampling_params) for output in outputs: print(f"Prompt: {output.prompt}") for i, out in enumerate(output.outputs): print(f"Sample {i + 1}: {out.text}")
This code efficiently generates multiple samples for the given prompt, leveraging vLLM's optimizations.
Benchmarking vLLM Performance
To truly appreciate the impact of vLLM, let's look at some performance comparisons:
Throughput Comparison
Based on the information provided, vLLM significantly outperforms other serving solutions:
– Up to 24x higher throughput compared to Hugging Face Transformers
– 2.2x to 3.5x higher throughput than Hugging Face Text Generation Inference (TGI)
Illustration:
“`
Throughput (Tokens/second)
|
| ****
| ****
| ****
| **** ****
| **** **** ****
| **** **** ****
|————————
HF TGI vLLM
“`
Memory Efficiency
vLLM's PagedAttention results in near-optimal memory usage:
– Only about 4% memory waste, compared to 60-80% in traditional systems
– This efficiency allows for serving larger models or handling more concurrent requests with the same hardware
Getting Started with vLLM
Now that we've explored the benefits of vLLM, let's walk through the process of setting it up and using it in your projects.
6.1 Installation
Installing vLLM is straightforward using pip:
!pip install vllm
6.2 Basic Usage for Offline Inference
Here's a simple example of using vLLM for offline text generation:
from vllm import LLM, SamplingParams # Initialize the model llm = LLM(model="meta-llama/Llama-2-13b-hf") # Prepare prompts prompts = [ "Write a short poem about artificial intelligence:", "Explain quantum computing in simple terms:" ] # Set sampling parameters sampling_params = SamplingParams(temperature=0.8, max_tokens=100) # Generate responses outputs = llm.generate(prompts, sampling_params) # Print the results for output in outputs: print(f"Prompt: {output.prompt}") print(f"Generated text: {output.outputs[0].text}\n")
This script demonstrates how to load a model, set sampling parameters, and generate text for multiple prompts.
6.3 Setting Up a vLLM Server
For online serving, vLLM provides an OpenAI-compatible API server. Here's how to set it up:
1. Start the server:
python -m vllm.entrypoints.openai.api_server --model meta-llama/Llama-2-13b-hf
2. Query the server using curl:
curl http://localhost:8000/v1/completions \ -H "Content-Type: application/json" \ -d '{ "model": "meta-llama/Llama-2-13b-hf", "prompt": "The benefits of artificial intelligence include:", "max_tokens": 100, "temperature": 0.7 }'
This setup allows you to serve your LLM with an interface compatible with OpenAI's API, making it easy to integrate into existing applications.
Advanced Topics on vLLM
While vLLM offers significant improvements in LLM serving, there are additional considerations and advanced topics to explore:
7.1 Model Quantization
For even more efficient serving, especially on hardware with limited memory, quantization techniques can be employed. While vLLM itself doesn't currently support quantization, it can be used in conjunction with quantized models:
from transformers import AutoModelForCausalLM, AutoTokenizer import torch # Load a quantized model model_name = "meta-llama/Llama-2-13b-hf" model = AutoModelForCausalLM.from_pretrained(model_name, device_map="auto", load_in_8bit=True) tokenizer = AutoTokenizer.from_pretrained(model_name) # Use the quantized model with vLLM from vllm import LLM llm = LLM(model=model, tokenizer=tokenizer)
7.2 Distributed Inference
For extremely large models or high-traffic applications, distributed inference across multiple GPUs or machines may be necessary. While vLLM doesn't natively support this, it can be integrated into distributed systems using frameworks like Ray:
import ray from vllm import LLM @ray.remote(num_gpus=1) class DistributedLLM: def __init__(self, model_name): self.llm = LLM(model=model_name) def generate(self, prompt, params): return self.llm.generate(prompt, params) # Initialize distributed LLMs llm1 = DistributedLLM.remote("meta-llama/Llama-2-13b-hf") llm2 = DistributedLLM.remote("meta-llama/Llama-2-13b-hf") # Use them in parallel result1 = llm1.generate.remote("Prompt 1", sampling_params) result2 = llm2.generate.remote("Prompt 2", sampling_params) # Retrieve results print(ray.get([result1, result2]))
7.3 Monitoring and Observability
When serving LLMs in production, monitoring is crucial. While vLLM doesn't provide built-in monitoring, you can integrate it with tools like Prometheus and Grafana:
from prometheus_client import start_http_server, Summary from vllm import LLM # Define metrics REQUEST_TIME = Summary('request_processing_seconds', 'Time spent processing request') # Initialize vLLM llm = LLM(model="meta-llama/Llama-2-13b-hf") # Expose metrics start_http_server(8000) # Use the model with monitoring @REQUEST_TIME.time() def process_request(prompt): return llm.generate(prompt) # Your serving loop here
This setup allows you to track metrics like request processing time, which can be visualized in Grafana dashboards.
Conclusion
Serving Large Language Models efficiently is a complex but crucial task in the age of AI. vLLM, with its innovative PagedAttention algorithm and optimized implementation, represents a significant step forward in making LLM deployment more accessible and cost-effective.
By dramatically improving throughput, reducing memory waste, and enabling more flexible serving options, vLLM opens up new possibilities for integrating powerful language models into a wide range of applications. Whether you're building a chatbot, a content generation system, or any other NLP-powered application, understanding and leveraging tools like vLLM will be key to success.
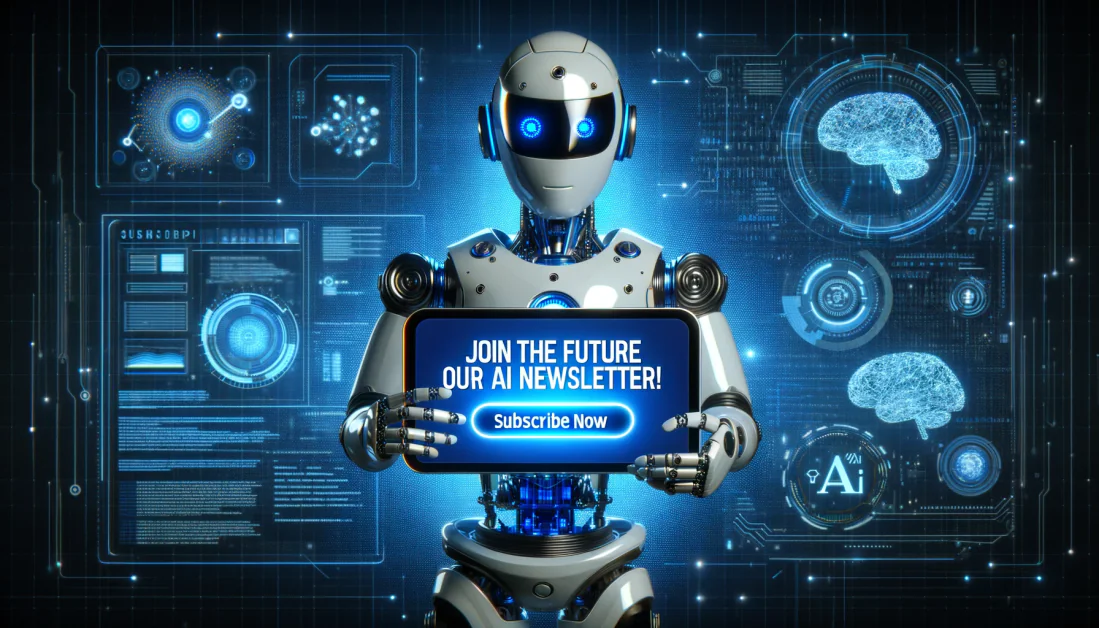
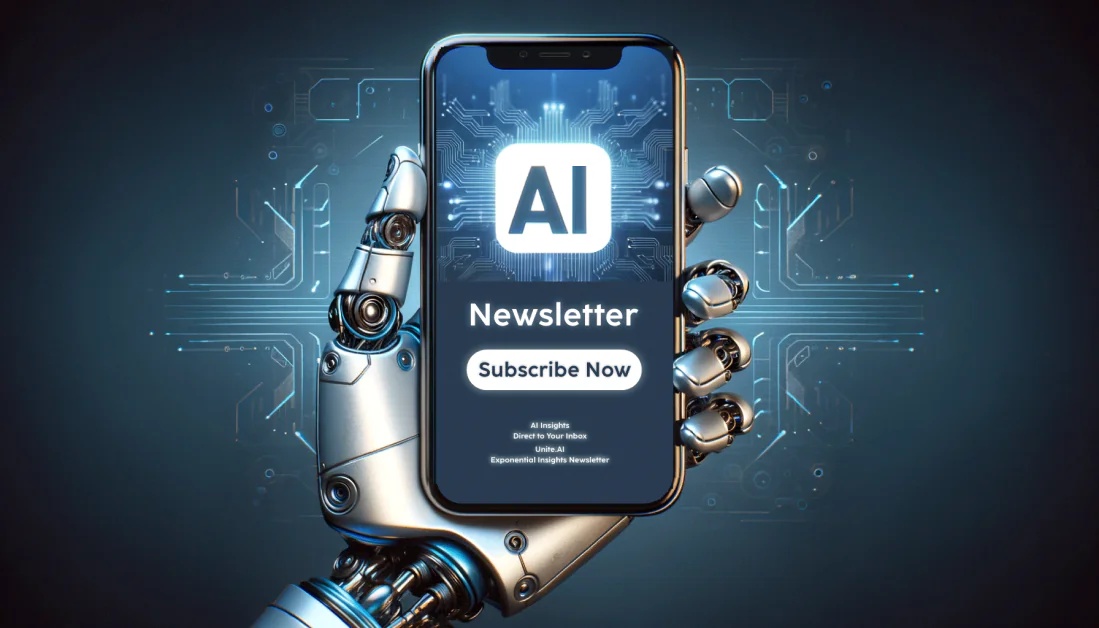